การจับคู่เทมเพลตเป็นเทคนิค โดยการจับคู่แพตช์หรือเทมเพลตจากรูปภาพจริง นี่เป็นกลไกการจับคู่รูปแบบโดยพื้นฐาน
ใน Python มีโมดูล OpenCV การใช้ openCV ทำให้เราสามารถค้นหาการจับคู่ได้อย่างง่ายดาย ดังนั้นในปัญหานี้จึงใช้เทคนิคการจับคู่เทมเพลต OpenVC
ในการใช้ฟังก์ชัน OpenCV เราจำเป็นต้องดาวน์โหลดโดยใช้ pip .
sudo pip3 install opencv-python
สำหรับงานจับคู่เทมเพลต มีปัจจัยความแม่นยำ ปัจจัยนี้เรียกว่าขีดจำกัด ตัวอย่างเช่น เราสามารถพูดได้ว่าเราสามารถสร้างรูปแบบการจดจำใบหน้าได้อย่างง่ายดายโดยใช้โซลูชันการจับคู่เทมเพลตนี้ เราสามารถจัดเตรียมรูปภาพของดวงตาหรือส่วนอื่น ๆ ของใบหน้า จากนั้นใช้รูปภาพเหล่านั้นเป็นเทมเพลต เพื่อค้นหาคู่ที่ตรงกันได้อย่างง่ายดาย แต่มีดวงตาที่แตกต่างกัน ดังนั้น หากเราตั้งค่าระดับความแม่นยำเป็น 50% ก็จะตรวจพบได้ดีกว่าระดับความแม่นยำ 100% โดยทั่วไป ระดับความแม่นยำจะอยู่ที่ 80% ในแต่ละกรณี
ขั้นตอนในการจับคู่เทมเพลต
-
ถ่ายภาพจริงแล้วแปลงเป็นภาพระดับสีเทา
-
นำเทมเพลตเป็นรูปภาพระดับสีเทา
-
เทมเพลตจะเลื่อนไปบนภาพจริงและค้นหาตำแหน่งที่ระดับความแม่นยำตรงกัน
-
เมื่อผลลัพธ์มากกว่าระดับความแม่นยำ ให้ทำเครื่องหมายตำแหน่งนั้นว่าตรวจพบ
สำหรับกรณีแรก รูปภาพอินพุตและเทมเพลตคือ −
ภาพหลัก
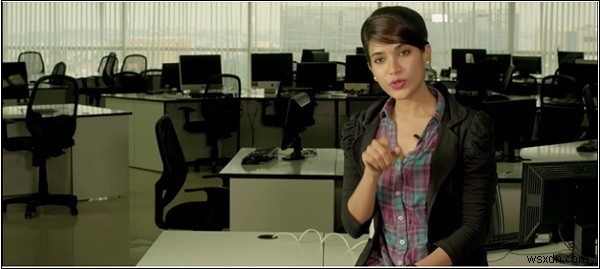
แม่แบบ

โค้ดตัวอย่าง
import cv2 import numpy as np #open the main image and convert it to gray scale image main_image = cv2.imread('main_image.png') gray_image = cv2.cvtColor(main_image, cv2.COLOR_BGR2GRAY) #open the template as gray scale image template = cv2.imread('template1.png', 0) width, height = template.shape[::-1] #get the width and height #match the template using cv2.matchTemplate match = cv2.matchTemplate(gray_image, template, cv2.TM_CCOEFF_NORMED) threshold = 0.8 position = np.where(match >= threshold) #get the location of template in the image for point in zip(*position[::-1]): #draw the rectangle around the matched template cv2.rectangle(main_image, point, (point[0] + width, point[1] + height), (0, 204, 153), 0) cv2.imshow('Template Found', main_image) cv2.waitKey(0)
ผลลัพธ์
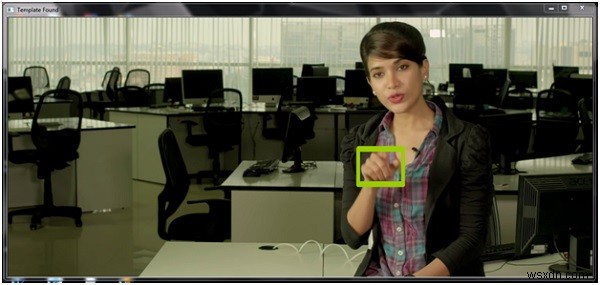
โค้ดด้านบนไม่รองรับการสเกลหลายขนาด ดังนั้นหากขนาดของเทมเพลตไม่เท่ากันก็จะตรวจไม่พบ ดังนั้นในตอนต่อไป เราจะมาดูกันว่าเราจะใช้ฟีเจอร์การปรับขนาดหลายขนาดเพื่อตรวจหาเทมเพลตได้อย่างไร
ในแนวทางนี้ รูปภาพจริงจะถูกแปลงเป็นขนาดต่างๆ กัน แต่ละครั้งที่ตรงกับรูปแบบ และหาค่าสัมประสิทธิ์สหสัมพันธ์ที่ใหญ่ที่สุดเพื่อค้นหาการจับคู่
รูปภาพจริงเหมือนกัน เทมเพลตอยู่ที่นี่ -
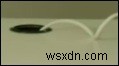
โค้ดตัวอย่าง
import imutils import cv2 import numpy as np #Open template and get canny template = cv2.imread('template3.jpg') template = cv2.cvtColor(template, cv2.COLOR_BGR2GRAY) template = cv2.Canny(template, 10, 25) (height, width) = template.shape[:2] #open the main image and convert it to gray scale image main_image = cv2.imread('main_image.png') gray_image = cv2.cvtColor(main_image, cv2.COLOR_BGR2GRAY) temp_found = None for scale in np.linspace(0.2, 1.0, 20)[::-1]: #resize the image and store the ratio resized_img = imutils.resize(gray_image, width = int(gray_image.shape[1] * scale)) ratio = gray_image.shape[1] / float(resized_img.shape[1]) if resized_img.shape[0] < height or resized_img.shape[1] < width: break #Convert to edged image for checking e = cv2.Canny(resized_img, 10, 25) match = cv2.matchTemplate(e, template, cv2.TM_CCOEFF) (_, val_max, _, loc_max) = cv2.minMaxLoc(match) if temp_found is None or val_max>temp_found[0]: temp_found = (val_max, loc_max, ratio) #Get information from temp_found to compute x,y coordinate (_, loc_max, r) = temp_found (x_start, y_start) = (int(loc_max[0]), int(loc_max[1])) (x_end, y_end) = (int((loc_max[0] + width)), int((loc_max[1] + height))) #Draw rectangle around the template cv2.rectangle(main_image, (x_start, y_start), (x_end, y_end), (153, 22, 0), 5) cv2.imshow('Template Found', main_image) cv2.waitKey(0)
ผลลัพธ์
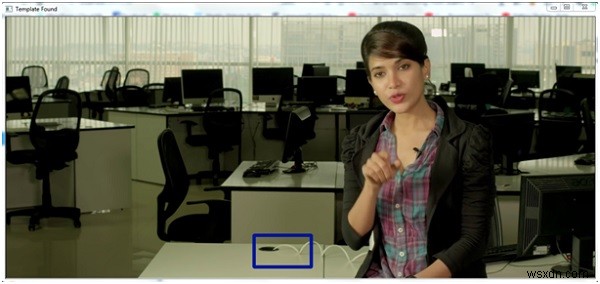